CSS Computer Science Past Paper 2022
PAPER-I (Subjective) 80 Marks
Attempt ONLY FOUR questions from PART-II. (20×4)
PART-II
SECTION-A
Q. No. 2.
(a) Using C++ write a function pattern (int n) that produces the following output. Pay special attention to the spaces and clearly state the return type of the function. Note that the following is just an example; your solution should work for any input “n”.
(b) Write the differences between Computer Science, Software Engineering, Information Technology, Information Systems, Computer Engineering, and Bioinformatics.
(c) Write the output of the following C++ code assuming that there is no error in the code:
int ary[4] = {1,2,3,4};
int *p1 = ary + 3;
cout << p1[-2] << endl;
int *p2 = &p1[-2];
*p2 = 10;
cout << ary[1] << endl;
Q. No. 3.
(a) Complete the C++ code below to find the number of distinct elements in an array in O(n) time (linear time complexity) where “n” is the number of elements in the array. For example, if the array is {3,1,3,8,2,1,8,2}, the number of distinct elements is 4 (to be returned from the function below) as the distinct elements are {1,2,3,8}. All the elements of the array are in the range [1,100]. Also, n >> 100 (n is significantly greater than 100).
int numDistinctElements(int[] array, int n)
{
int count = 0;
// write your code here
return count;
}
(b) Write a detailed note on the principles of Information Security/ Privacy.
(c) Write the output of the following C++ code assuming that there is no error in the code:
int v1 = 55;
int* p1 = &v1;
int* p2;
p2 = p1;
*p1 = 20;
cout << *p2 << endl;
cout << v1 << endl;
Q. No. 4.
(a) Write a C++ program to check whether a string is palindrome or not. A palindrome is a string, which when read in both forward and backward ways is the same, e.g., “radar”, “madam” etc. Note that these are only examples, you will take the string as an input from a user using the cin.getline
function and your code should be general that can work on any input string. You are not allowed to use any string-related library function.
(b) Write the output of the following C++ code assuming that there is no error in the code:
#include <iostream.h>
int main() {
int a = 12, b = 25;
cout << "a = " << a << endl;
cout << "b = " << b << endl;
cout << "a | b = " << (a | b) << endl;
return 0;
}
SECTION-B
Q. No. 5.
(a) Define a class called Circle using C++. An object of the class Circle can be created using any of the following ways (implement all the ways):
- By default, the circle will be of radius 1 inch and centered at (0,0).
- With a given value for radius, centered at (0,0).
- By providing the center coordinates and the radius is assumed to be 1.
- By providing the radius and the coordinates for the center.
- By providing another Circle object to initialize.
In the main function (driver program), separately create 5 different objects of the Circle class using a different way of object creation (mentioned above) for each of them.
(b) What is a virtual function in C++? Using an example, explain how the virtual function mechanism works.
Q. No. 6.
(a) Complete the following recursive function using C++ to calculate k raised to the power n, i.e., k^n. Use of any built-in (library) function is not allowed.
int power(int k, int n)
{
// write your code here
}
(b) Compare and contrast the DES, AES, and RSA encryption algorithms.
(c) Write the time complexity of the following C++ code in terms of Big-O notation. Assume that there is no error in the code:
int isPrime (unsigned int n)
{
for (unsigned int i = 2; (i * i) < n; i++)
{
// if i is a factor, then not prime
if (n % i == 0)
return 0;
}
// if we end loop without finding factor then number must be Prime
return 1;
}
Q. No. 7.
(a) The following function performs binary search on a given array of n numbers:
1. int binarySearch (int x, int v[], int n)
2. {
3. int low, high, mid;
4. low = 0;
5. high = n – 1;
6. while (low < = high)
7. {
8. mid = (low + high )/2;
9. if (x < v[mid])
10. high = mid – 1;
11. else if (x > v[mid])
12. low = mid + 1 ;
13. else return mid;
14. }
15. return -1;
16. }
Draw the control flow graph for the above function. Also, find its cyclomatic complexity.
(b) What is the difference between white-box (glass-box) testing and black-box testing? Which of these testing techniques helps in identifying more errors in a system under evaluation? Explain.
(c) Requirements can be specified using natural language specification. Write one user requirement and one system requirement for the following function: Automatically highlight incorrect spelling mistakes in an MS Word document.
Q. No. 8.
(a) Draw a finite automaton over ∑ = {0, 1} that accepts all binary strings starting and ending with 0 (single-0 string counts).
(b) Write a regular expression over ∑ = {0, 1} to represent a set of strings that begin with 101 and end with 110.
(c) Write a Context-Free Grammar (CFG) over ∑ = {0, 1} for the language of all binary strings of the form 0a 1b 0c where a + c = b.
PAPER–II (Subjective) 80 Marks
Attempt ONLY FOUR questions from PART-II. (20×4)
PART-II
SECTION-A
Q. 2.
(a) The following processes are being scheduled using a preemptive, round robin scheduling algorithm. Each process is assigned a numerical priority, with a higher number indicating a higher relative priority. In addition to the processes listed below, the system also has an idle task (which consumes no CPU resources and is identified as Pidle). This task has priority 0 and is scheduled whenever the system has no other available processes to run. The length of a time quantum is 10 units. If a process is preempted by a higher-priority process, the preempted process is placed at the end of the queue. Show the scheduling order of the processes using a Gantt chart. What is the turnaround time and waiting time for each process?
(b) Suppose the disk head is initially located at track 20, set to move in order of increasing tracks. Assume a disk with 100 tracks and that the disk request queue has random requests in it. The requested tracks, in the order received by the disk scheduler, are 18, 25, 73, 46, 9, 92. Using C-SCAN algorithm, find the performance in terms of average seek time.
(c) What design and management issues are raised by the existence of concurrency?
Q. 3.
(a) Consider the following snapshot of the system.
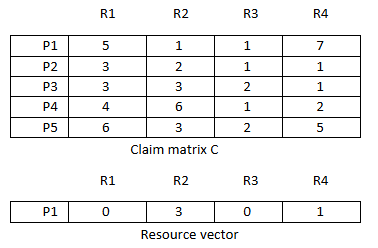
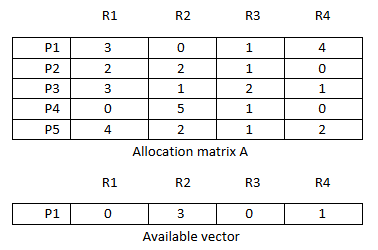
Using banker’s algorithm, determine whether the state is safe or unsafe. If the state is safe, illustrate the order in which the processes may complete. Otherwise, illustrate why the state is unsafe.
(b) Give an account of different functions of Logical File System.
(c) What resources are used when a thread is created? How do they differ from those used when a process is created?
Q. 4.
(a) Assume a pipeline with four stages: fetch instruction (FI), decode instruction and calculate addresses (DA), fetch operand (FO), and execute (EX). Draw a diagram for a sequence of 7 instructions, in which the third instruction is a branch that is taken and in which there are no data dependencies.
(b) A set associative cache consists of 64 lines, or slots, divided into 4-slot sets. Main memory contains 4K blocks of 128 words each. Show the format of main memory addresses.
(c) Why is DMA access to main memory given higher priority than CPU access to main memory?
Q. 5.
(a) Describe any one of the routing algorithms in detail, which is used to resolve the conflict between path selections.
(b) A company occupies four buildings on a campus. Each of these buildings has a 100 base T network running to all the floors. The buildings form a square 100 meters on each side. The network must support 100 Mbps data transfer rate. What will be the proposed design solution under the given condition?
(c) What is the main fault in TCP 3-way handshake which makes it a boon for attackers? Many operating systems generally associate a “Backlog” parameter with a listening socket that sets cap on the number of TCBs simultaneously in SYN-RECEIVED state. Does this parameter solve the problem in TCP 3-way handshake?
SECTION-B
Q. 6.
(a) Find the reflection, , of each of the following Structuring Elements (SE). The dot indicates the origin of the SE.
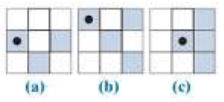
(b) The R, G, and B component images of an RGB image have the horizontal intensity profiles shown in the following diagram. Show your working to identify the color that a person would see in the middle column of this image.
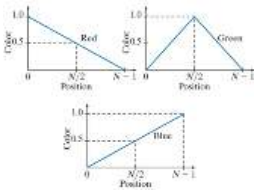
(c) How Digital Image Processing and Computer Vision are related? Discuss.
Q. 7.
(a) Explain 3-tier web application architecture.
(b) Write the jQuery code to slide elements up and down. Also, write the code to fade the elements in and out of visibility. Use HTML, CSS, and jQuery.
(c) What is web application promotion? Discuss some of the common webvertising methods.
Q. 8.
(a) Explain process of Web Application Testing.
(b) Explain in detail the Document Object Model (DOM). Also discuss XML and RSS.
(c) Explain how can you access a database from a JSP page? Give the database connectivity issues in detail.